Random Array Sampling¶
This plugin provides various blueprint nodes for generating random samples, possibly weighted, from a source array. Arrays can be of any type, with relative weights attached to favour sampling some items over others. A C++ template function is also provided for use in C++ source code.
Blueprint Nodes¶
Random Sample¶
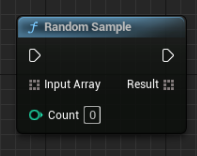
Get a random sample of Count items from the Input Array. If there are fewer than Count items in the input array, the function will return all items in the input array in a random order.
Random Sample From Stream¶
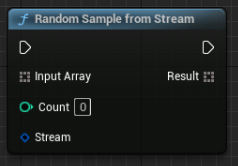
Like Random Sample but using a custom random stream.
Weighted Sample¶
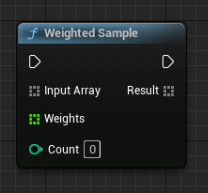
Get a random sample of Count items from the Input Array. Each item in the Input Array has a weight corresponding to the bias towards sampling that item relative to all the other item weights. There must be at least as many weights in the Weights array as there are items in the Input Array.
Weighted Sample From Stream¶
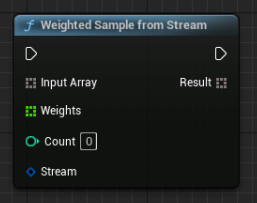
Like Weighted Sample but using a custom random stream.
Weighted Shuffle¶
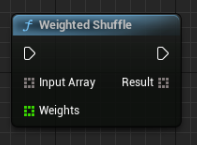
Shuffle the Input Array, returning the entire contents in a different order. Each item in the Input Array has a weight corresponding to the bias towards sampling that item relative to all the other item weights. There must be at least as many weights in the Weights array as there are items in the Input Array.
The result of this function is similar to calling Weighted Sample with the Count equal to the number of items in the Input Array.
Weighted Shuffle From Stream¶
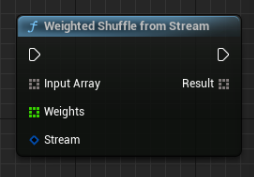
Like Weighted Shuffle but using a custom random stream.
C++ Function¶
Include: “RandomSample.h”
Namespace: RandomShuffles
-
template<typename It, typename Wt, typename Out, typename Rand>
Out RandomSample(It begin, It end, Wt wbegin, Out out, std::size_t count, Rand randFunc)¶
Randomly select count
items from the sequence [begin, end)
, using relative weights in the sequence starting at wbegin
. Samples will be written in sequence to out
. The given random number generator, randFunc
, will be used as the source of randomness.
Template Parameters¶
It An input iterator type.
Wt An input iterator type with a value type which is convertible to
float
.Out An output iterator type for which the statement
*out = *in
is well-formed, whereout
is of typeOut
andin
is of typeIt
.Rand A callable type with the signature
Rand(float, float)
.
Parameters¶
begin An input iterator denoting the first item in the sequence to sample from.
end An input iterator one past the end of the sequence to sample from.
wbegin An input iterator denoting the first weight in the sequence of relative weights.
out An output iterator denoting the position in the output sequence to start writing to.
count The number of items to sample.
randFunc A random number generator function.
Returns¶
One past the end of the last output element written to the output sequence, or out
if no elements were written.
Notes¶
The weight sequence must contain at least as many elements as the input sequence.
The output sequence must be able to write at min(count, std::distance(begin, end))
elements.
If count == 0
or begin == end
, no elements are written.
The randFunc
parameter accepts a minimum and maximum value and should return a random number between these values.